목록전체 글 (956)
넘치게 채우기
입출력 import sys sys.stdin.readline() # 한줄 전체 입력받기. input()보다 빠르게 입력받을 수 있다. 개행문자 \n도 딸려오니 주의. a, b, c = map(int, sys.stdin.readline().split()) # a, b, c에 각각 넣기 형변환 int(x) # int로 변환 str(x) # 문자열로 변환 float(x) # 실수 타입 변환 chr(x) # 문자로 변환 bool(x) # 참/거짓값으며 변환(0이나 null이 아닌경우 모두 True) 수학 1e9 = 10^9 2e9 = 2 * 1e9 (INT_MAX에 거의 근접한 값이다) 1e9 + 7 # 보통 온라인 저지 사이트나 알고리즘 대회에서 값이 너무 커질 때, 오버플로우를 막기 위해서 1e9 + 7로 ..
https://leetcode.com/problems/longest-substring-without-repeating-characters/description/?envType=study-plan-v2&envId=top-interview-150 int: n = len(s) maxLen = 0 left = 0 charSet = set() for right in range(n): while s[right] in charSet: charSet.remove(s[left]) left += 1 charSet.add(s[right]) maxLen = max(maxLen, right - left + 1) return maxLen Java class Solution { public int lengthOfLongestSub..
https://leetcode.com/problems/excel-sheet-column-title/description/ Excel Sheet Column Title - LeetCode Can you solve this real interview question? Excel Sheet Column Title - Given an integer columnNumber, return its corresponding column title as it appears in an Excel sheet. For example: A -> 1 B -> 2 C -> 3 ... Z -> 26 AA -> 27 AB -> 28 ... Example 1: I leetcode.com 문제 유형 : 문자열 처리 문제 난이도 : Eas..
https://leetcode.com/problems/repeated-substring-pattern/ Repeated Substring Pattern - LeetCode Can you solve this real interview question? Repeated Substring Pattern - Given a string s, check if it can be constructed by taking a substring of it and appending multiple copies of the substring together. Example 1: Input: s = "abab" Output: true Expl leetcode.com 문제 유형 : 문자열 처리 문제 난이도 : Easy 문제 Giv..
https://leetcode.com/problems/minimum-size-subarray-sum/description/?envType=study-plan-v2&envId=top-interview-150 Minimum Size Subarray Sum - LeetCode Can you solve this real interview question? Minimum Size Subarray Sum - Given an array of positive integers nums and a positive integer target, return the minimal length of a subarray whose sum is greater than or equal to target. If there is no s..
https://leetcode.com/problems/3sum/description/?envType=study-plan-v2&envId=top-interview-150 3Sum - LeetCode Can you solve this real interview question? 3Sum - Given an integer array nums, return all the triplets [nums[i], nums[j], nums[k]] such that i != j, i != k, and j != k, and nums[i] + nums[j] + nums[k] == 0. Notice that the solution set must not contain du leetcode.com 문제 유형 : 투 포인터 문제 난..
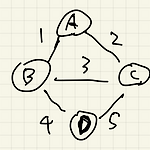
최소 신장 트리 (Minimum Spanning Tree, MST) 신장 트리는 그래프의 모든 정점을 연결하는 트리를 말한다. (사이클이 생기지 않음) 최소 신장 트리는 그래프의 모든 정점을 연결하는 간선들의 가중치의 합이 최소인 트리를 말한다. (신장 트리중 가장 저렴한 비용) 크루스칼 알고리즘(Kruscal's Algorithm) 크루스칼 알고리즘은 최소 신장 트리를 찾는 방법 중 하나이다. 1. 모든 간선들을 가중치 기준으로 오름차순 정렬한다. 2. 가장 작은 가중치의 간선부터 선택한다. 3. 고른 간선이 사이클을 형성한다면, 선택하지 않는다. 4. 2와3을 계속 반복한다. 아래는 크루스칼 알고리즘의 예시이다. 우선, 간선들의 가중치를 기준으로 오름차순 정렬해준다. A-B : 1 A-C : 2 B-..
https://leetcode.com/problems/maximal-network-rank/description/ Maximal Network Rank - LeetCode Can you solve this real interview question? Maximal Network Rank - There is an infrastructure of n cities with some number of roads connecting these cities. Each roads[i] = [ai, bi] indicates that there is a bidirectional road between cities ai and bi. The leetcode.com 문제 유형 : 그래프 문제 난이도 : Medium 문제 T..
https://leetcode.com/problems/01-matrix/description/ 01 Matrix - LeetCode Can you solve this real interview question? 01 Matrix - Given an m x n binary matrix mat, return the distance of the nearest 0 for each cell. The distance between two adjacent cells is 1. Example 1: [https://assets.leetcode.com/uploads/2021/04/24/01-1-g leetcode.com 문제 유형 : BFS / DFS (+Multi-Source BFS, 다중 출발점 BFS) 문제 난이도 ..
https://leetcode.com/problems/sliding-window-maximum/description/ Sliding Window Maximum - LeetCode Can you solve this real interview question? Sliding Window Maximum - You are given an array of integers nums, there is a sliding window of size k which is moving from the very left of the array to the very right. You can only see the k numbers in the wind leetcode.com 문제 유형 : 슬라이딩 윈도우 문제 난이도 : Har..