목록알고리즘 (285)
넘치게 채우기
https://leetcode.com/problems/magnetic-force-between-two-balls/description/leetcode - Magnetic Force Between Two Balls문제 유형 : 이진 탐색문제 난이도 : Medium 문제In the universe Earth C-137, Rick discovered a special form of magnetic force between two balls if they are put in his new invented basket. Rick has n empty baskets, the ith basket is at position[i], Morty has m balls and needs to distribute the bal..
https://leetcode.com/problems/sequential-digits/description/ LeetCode - The World's Leading Online Programming Learning Platform Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com Leetcode - Sequential Digits 문제 유형 : 구현 문제 난이도 : Medium 문제 An integer has sequential digits if and only if each di..
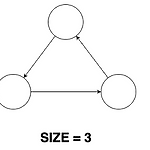
https://school.programmers.co.kr/learn/courses/30/lessons/258711 프로그래머스 코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요. programmers.co.kr 프로그래머스 - 도넛과 막대 그래프(2024 KAKAO WINTER INTERNSHIP) 문제 유형 : 그래프 문제 난이도 : Level 2 문제 도넛 모양 그래프, 막대 모양 그래프, 8자 모양 그래프들이 있습니다. 이 그래프들은 1개 이상의 정점과, 정점들을 연결하는 단방향 간선으로 이루어져 있습니다. 크기가 n인 도넛 모양 그래프는 n개의 정점과 n개의 간선이 있습니다. 도넛 모양 그래프의..
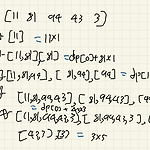
https://leetcode.com/problems/sum-of-subarray-minimums/description/?envType=daily-question&envId=2024-01-20 LeetCode - The World's Leading Online Programming Learning Platform Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com LeetCode - Sum of Subarray Minimums 문제 유형 : 다이나믹 프로그래밍, 단조 스택 문제 난이..
https://leetcode.com/problems/unique-number-of-occurrences/description/ LeetCode - The World's Leading Online Programming Learning Platform Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com leetcode - Unique Number of Occurrences 문제 유형 : 해시 문제 난이도 : Easy 문제 Given an array of integers arr, ret..
https://leetcode.com/problems/determine-if-string-halves-are-alike/description/ Determine if String Halves Are Alike - LeetCodeCan you solve this real interview question? Determine if String Halves Are Alike - You are given a string s of even length. Split this string into two halves of equal lengths, and let a be the first half and b be the second half. Two strings are alike if tleetcode.comlee..
https://leetcode.com/problems/maximum-difference-between-node-and-ancestor/description/ Maximum Difference Between Node and Ancestor - LeetCode Can you solve this real interview question? Maximum Difference Between Node and Ancestor - Given the root of a binary tree, find the maximum value v for which there exist different nodes a and b where v = |a.val - b.val| and a is an ancestor of b. A node..
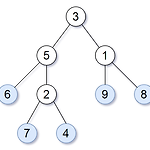
https://leetcode.com/problems/leaf-similar-trees/description/ Leaf-Similar Trees - LeetCode Can you solve this real interview question? Leaf-Similar Trees - Consider all the leaves of a binary tree, from left to right order, the values of those leaves form a leaf value sequence. [https://s3-lc-upload.s3.amazonaws.com/uploads/2018/07/16/tree.png leetcode.com leetcode - Lear-Similar Trees 문제 유형 : ..
https://leetcode.com/problems/range-sum-of-bst/description/ Range Sum of BST - LeetCode Can you solve this real interview question? Range Sum of BST - Given the root node of a binary search tree and two integers low and high, return the sum of values of all nodes with a value in the inclusive range [low, high]. Example 1: [https://assets.l leetcode.com leetcode - Range Sum of BST 문제 유형 : bfs/dfs..
https://leetcode.com/problems/arithmetic-slices-ii-subsequence/description/ Arithmetic Slices II - Subsequence - LeetCode Can you solve this real interview question? Arithmetic Slices II - Subsequence - Given an integer array nums, return the number of all the arithmetic subsequences of nums. A sequence of numbers is called arithmetic if it consists of at least three elements leetcode.com leetco..